Introduction
Over the past 20 years, Python has gained popularity as an open-source, cross-platform programming language. The first edition came out in 1991. Version 3.12.4 is the most recent. The Python programming language's reference implementation is known as CPython. The most popular and default implementation of the language, CPython, is written in C. Python's many extensions make it a versatile programming language; examples include web development (using the Django Web framework, for example), simulations, scientific computation and calculations, etc.
The Python Software Foundation (https://www.python.org) is the source of the language's maintenance and availability. The Python language interpreter and a simple code editor, known as an integrated development environment, or IDLE, are both available for download here. Python typically requires additional features to complete your tasks. After that, using and installing other Python packages made by outside developers is required. These packages must be downloaded and installed individually. You can either use a distribution package like Anaconda or something called PIP.
Although Python is an object-oriented programming language (OOP), we can use it for simple applications without having to be aware of or make use of its object-oriented capabilities. Due to Python's interpretive nature, developers create Python (.py) files in a text editor and then load those files into the interpreter to run the code. This can be done automatically by the editor you are using, or it can be done manually.
First Python program
Let start with first python program
Open Python Editor and type the following:
print (“Hello World! “)
Python Shell
Use the Python Shell in interactive mode as seen below.
In the Python Shell, type each command one at a time following the ">>>" symbol.
>>> print (“Hello World! “)
"Interactive Python interpreter session, also known as the Python shell," is indicated by the prompt >>> on the final line. The typical terminal command prompt is not like this one!


Python Scripting Mode
We can write a Python program with several Python commands in "Scripting" mode and save it as a file (.py).
Use the Python IDLE to run scripts in Python.
We can choose File-New File from the Python Shell, or File-Open to launch an already-running Python script or program.
Let's write the following in a new script that we create:



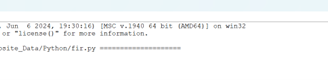
First Basic Python Programming
Variables
A variable can have a short name or single character (like x and y) or a more descriptive name.
Use of the assignment operator "=" defines variables. Python is dynamically typed, which allows variables to have their types changed and assigned without having to declare them. Values can originate from function output, constants, or calculations involving the values of other variables.
Creating and using Variables in Python
Enter the following using the standard IDLE (or another Python editor):
Example 01: Write a Program to Multiply any two Integers and display the result?
x = 2
y = 3
z=x * y
print(z)
Result
6
A Variable can be declare the number in any format such as integer, float or complex number
Example 02 : Write a Program to find the result for the given equation y = a*x + b, where a is integer, b is float and c is a complex numbers?
a=5 #integer
x=3.2 #float
b=3+2j #comples
y=a*x+b
print(y)
Result
19+2j
Strings
In Python, strings are enclosed in either single or double quotation marks. "Hello" and "Hello" are the same. The print function can be used to output strings to the screen. Take print("Hello") as an example.
Example 03 : Write a Program to print the string in different format such single character, lower case, length, replace character, split character?
a = "Hello Muceetech!"
print(a)
print(a[0])
print(a[0:11])
print(len(a))
print(a.lower( ))
print(a.upper( ))
print(a.replace("H","F"))
print(a.split(" "))
Result
Hello Muceetech!
H
Hello Mucee
16
hello muceetech!
HELLO MUCEETECH!
Fello Muceetech!
['Hello', 'Muceetech!']
Example 04: Write a Program to get the input from user and display the result.
print( "Enter your name : " )
x = input ( )
print( "Hello," +x )
Result
Enter your name :
MUCEETECHOLOGIES
Hello,MUCEETECHOLOGIES